Getting Started
How do I spawn a Projectile?
Call the ProjectileCaller from any of your scripts and request any of its Projectile Resources.
extends Node2D
@onready var projectile_caller = $ProjectileCaller2D
func _process(_delta):
if Input.is_mouse_button_pressed(MOUSE_BUTTON_LEFT):
var destination = get_global_mouse_position()
projectile_caller.request_projectile(0, position, destination)
How do I create a Projectile Blueprint?
Right click on any Godot FileSystem folder Create New Resource
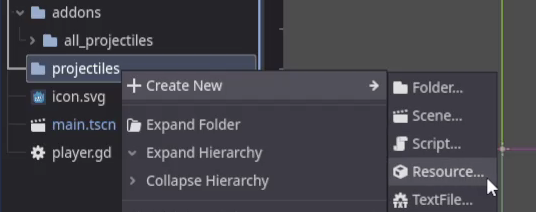
How do I modify the behavior of a projectile?
You can change the behavior of your Projectiles when requesting its creation from the ProjectileCaller.
The request_projectile method will take your custom functions as parameters.
extends Node2D
@onready var projectile_caller = $ProjectileCaller2D
# In this example the "custom_movement" function is passed as a Callable in the optional "move_method" parameter.
# The ProjectileCaller "request_projectile" method can also change the collision, expiration or target of your projectiles.
func _process(_delta):
if Input.is_mouse_button_pressed(MOUSE_BUTTON_LEFT):
var destination = get_global_mouse_position()
projectile_caller.request_projectile(0, position, destination, null, custom_movement)
# Each optional Callable argument follows a define pattern and must take a set number of parameters.
# To learn more about these custom methods and how to use them go to the ProjectileCaller section.
func custom_movement(proj: Projectile2D, _delta: float) -> Vector2:
return Vector2.UP